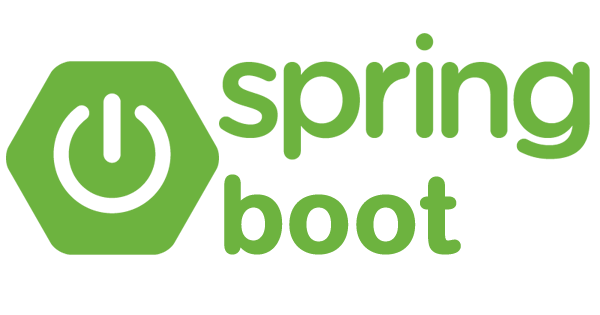
사용자 페이지
사용자의 경우 레스토랑을 관리하지 않으므로 레스토랑 정보를 추가하거나 수정할필요는 없고 조회하는 기능만 있으면 된다.
- 모든 Restaurant정보 가져오기
- 특정 Restaurant정보 가져오기
Request Parameter를 통한 레스토랑 조회 control
요청시 RequestParameter
를 통해 원하는 지역, 카테고리를 통해 해당 조건을 만족하는 레스토랑 정보를 모두 가져올 수 있도록 한다. 특정 Restaurant 정보를 가져오는 함수들은 관리자에서 만들었던 내용과 같으므로 생각한다.
@CrossOrigin @RestController @RequiredArgsConstructor public class RestaurantController { private final RestaurantService restaurantService;
@GetMapping("/restaurants") public List<Restaurant> list(@RequestParam("region") String region, @RequestParam("category") Long category) { List<Restaurant> restaurants = restaurantService.getRestaurants(region, category); return restaurants; }
@GetMapping("/restaurants/{id}") public Restaurant detail(@PathVariable Long id) throws Exception { Restaurant restaurant = restaurantService.getRestaurant(id); return restaurant; } }
|
Request Parameter를 통한 레스토랑 조회 테스트 코드 작성
@Test public void RequestParameter를_이용한_list를_확인한다() throws Exception { List<Restaurant> restaurants = new ArrayList<>(); restaurants.add(Restaurant.builder() .id(1004L) .categoryId(1L) .name("JOKER House") .address("Seoul") .build());
given(restaurantService.getRestaurants("Seoul", 1L)).willReturn(restaurants);
ResultActions resultActions = mockMvc.perform(get("/restaurants?region=Seoul&category=1"));
resultActions .andExpect(status().isOk()) .andExpect(content().string(containsString("\"id\":1004"))) .andExpect(content().string(containsString("\"name\":\"JOKER House\""))) .andDo(print()); }
|
Service에서 Region과 CategoryId를 이용한 조회 메소드 구현
control로부터 받은 region과 categoryId를 갖고 해당 조건을 만족하는 레스토랑 정보를 가져와 반환한다. 만약 레스토랑 정보가 없다고 하면 RestaurantNotFoundException
예외를 일으킨다.
@Service @RequiredArgsConstructor public class RestaurantService { private final RestaurantRepository restaurantRepository;
public List<Restaurant> getRestaurants(String region, long categoryId) { List<Restaurant> restaurants = restaurantRepository.findAllByAddressContainingAndCategoryId(region, categoryId); return restaurants; }
public Restaurant getRestaurant(Long id) { Optional<Restaurant> optional = restaurantRepository.findById(id);
if (optional.isPresent()) { Restaurant restaurant = optional.get(); return restaurant; }else{ throw new RestaurantNotFoundException(id); } } }
|
public List<Restaurant> getRestaurants(String region, long categoryId) { List<Restaurant> restaurants = restaurantRepository.findAllByAddressContainingAndCategoryId(region, categoryId);
return restaurants; }
|
Repository에 method추가
@Repository public interface RestaurantRepository extends JpaRepository<Restaurant, Long> { List<Restaurant> findAllByAddressContainingAndCategoryId(String region, long categoryId); }
|