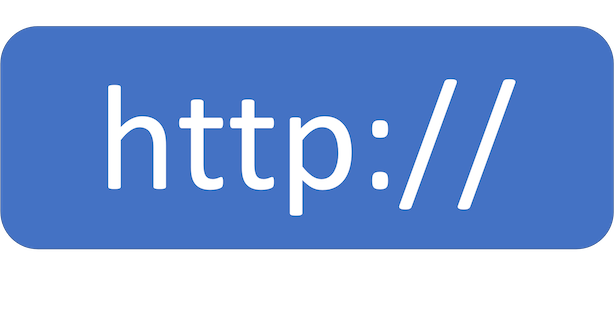
HttpClient 사용하기
HttpClient 는 Apache에서 제공하는 라이브러리로 HTTP 를 사용하는 통신에 손쉽게 자원을 요청하고 응답받을 수 있게 도와준다.
implementation group: 'org.apache.httpcomponents', name: 'httpclient', version: '4.3.4'
|
Back-end Controller
HttpClient 를 사용해 요청 테스트를 진행하기 위한 서버 Controller를 생성한다.
@RestController public class HttpClientBackend {
@PostMapping("/hello") @CrossOrigin(origins = "*", allowedHeaders = "*") public String hello(){ return "Hello World!"; } }
|
HttpClient 객체 가져오기
- HttpClient 객체를 가저오는 방법은 HttpClients 클래스에서 제공하는 createDefault 메소드를 이용하는 방법과 HttpClientBuilder 를 이용한 방법이 있다.
- createDefault 내부를 보면 HttpClientBuilder 를 이용해 HttpClient 객체를 가져오기 때문에 사실상 똑같다.
CloseableHttpClient client = HttpClients.createDefault(); CloseableHttpClient client2 = HttpClientBuilder.create().build();
|
Post 요청을 위한 HttpPost 객체 생성
- HttpPost를 이용해 Post 요청보내기 위한 요청 객체를 생성한다.
- 객체를 만들때 요청을 보내기 위한 요청 URL을 넣어준다.
HttpPost httpPost = new HttpPost("http://localhost:8080/hello");
|
요청 후 응답 객체 받아오기
- HttpClient 객체의 execute 메소드를 이용해 서버 측으로 요청을 보낸다.
- execute 메소드의 매게변수로 생성한 요청 객체 (HttpGet, HttpPost, HttpPut, HttpPatch) 를 넣어준다.
CloseableHttpResponse response = client.execute(httpPost);
|
응답 값 가져오기
- 응답 객체로부터 입력 스트림 을 가져와 값을 읽어온다.
BufferedReader br = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
|
전체 소스 코드
@SpringBootApplication public class JavaHttpclientApplication {
public static void main(String[] args) throws IOException { CloseableHttpClient client = HttpClients.createDefault(); HttpPost httpPost = new HttpPost("http://localhost:8080/hello"); CloseableHttpResponse response = client.execute(httpPost);
System.out.println("============================ body =============================");
String inputLine; StringBuffer result = new StringBuffer(); BufferedReader br = new BufferedReader(new InputStreamReader(response.getEntity().getContent()));
while ((inputLine = br.readLine()) != null) { result.append(inputLine); }
System.out.println(result);
System.out.println("============================ body =============================");
br.close(); client.close(); } }
|
HttpClient Fluent API 사용하기
Fluent API 를 이용하면 조금더 쉽고 간편하고 직관적으로 HTTP 요청을 할 수 있다.
라이브러리 추가
implementation group: 'org.apache.httpcomponents', name: 'httpclient', version: '4.3.4'
implementation group: 'org.apache.httpcomponents', name: 'fluent-hc', version: '4.3.4' }
|
서버측에 요청하고 응답 받기
Fluent API 에서 제공하는 Request 를 이용하면 요청 객체를 따로 생성할 필요 없이 간편하게 요청하고 실행해 응답값을 받아올 수 있다.
HttpResponse response = Request .Post("http://localhost:8080/hello") .execute() .returnResponse();
|
전체 소스 코드
public class JavaHttpClientFluentApi { public static void main(String[] args) throws IOException { HttpResponse response = Request .Post("http://localhost:8080/hello") .execute() .returnResponse();
String line; StringBuilder stringBuilder = new StringBuilder(); BufferedReader br = new BufferedReader(new InputStreamReader(response.getEntity().getContent())); while((line = br.readLine()) != null){ stringBuilder.append(line); }
System.out.println("=========================== start ============================"); System.out.println("Status Code: " + response.getStatusLine().getStatusCode()); System.out.println("Content: " + stringBuilder.toString()); System.out.println("============================ end ============================="); } }
|
참고 사이트
https://www.baeldung.com/httpclient4