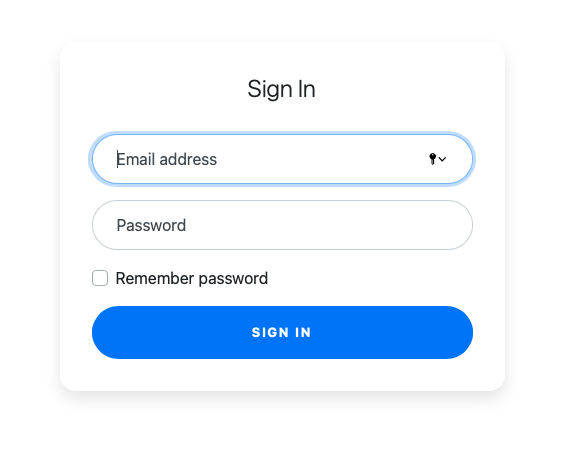
Spring properties 설정하기
Spring Security에서 사용하는 기본적인 user정보를 등록한다. jpa를 사용하므로 관련 옵션도 추가해준다.
application.yml
spring: security: user: name: user password: 1234 jpa: hibernate: ddl-auto: create-drop properties: hibernate: show_sql: true format_sql: true profiles: include: mysql
logging: level: org.springframework.security: DEBUG
|
application-mysql.yml
spring: datasource: driver-class-name: com.mysql.cj.jdbc.Driver url: jdbc:mysql://localhost:3306/{테이블명}?serverTimezone=UTC&characterEncoding=UTF-8 username: user_id password: user_password
jpa: database: mysql database-platform: org.hibernate.dialect.MySQL5InnoDBDialect
|
Entity 생성하기
사용자 정보를 저장하기 위한 Account Entity를 만든다.
@Entity @NoArgsConstructor @AllArgsConstructor @Getter public class Account {
@Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long idx; private String username;
@Column(unique = true, nullable = false) private String email;
private String password;
@Enumerated(EnumType.STRING) private Role role;
private String getRoleValue(){ return this.role.getValue(); }
@Builder public Account(String username, String email, String password, Role role){ this.username = username; this.email = email; this.password = password; this.role = role; } }
|
권한을 지정하기위한 Role
public enum Role { USER("ROLE_USER"), ADMIN("ROLE_ADMIN");
private String value; Role(String value){ this.value = value; }
public String getValue(){ return this.value; } }
|
DB로부터 사용자 정보를 가져오기 위한 Repository
@Repository public interface AccountRepository extends JpaRepository<Account, Long> { public Optional<Account> findByEmail(String email);}
|