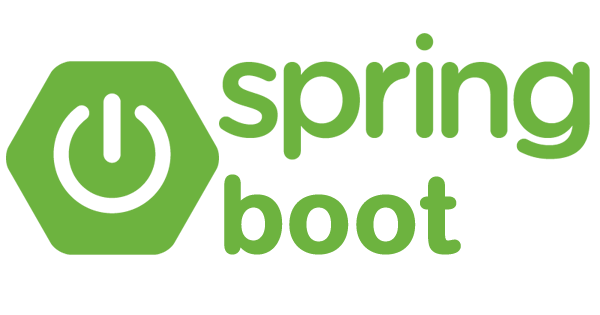
2. 특정 레스토랑에 대한 정보 가져오기
특정가게에 대한 상세정보 요청을 처리하기 위한 control 추가
특정 레스토랑의 정보를 가져오고 싶을 경우 restaurant의 Id값을 이용할 수 있다. /restaurant
경로에 사용자가 원하는 restaurantId 를 더해 해당 레스토랑에 대한 정보를 반환하도록 한다.
@GetMapping("/restaurants/{id}") public Restaurant detail(@PathVariable Long id) throws Exception { Restaurant restaurant = restaurantService.getRestaurant(id); return restaurant; }
|
테스트 코드 작성
특정 id값으로 갖는 restaurant에 대한 정보를 가져오는 테스트를 구현한다. 요청이 정상적으로 진행된 경우에는 Status으로 200과 함께 해당 레스토랑에 대한 정보를 받을 수 있다.
@Test public void 특정_가게_상세정보를_가져온다() throws Exception { Restaurant restaurant = Restaurant.builder() .id(1004L) .categoryId(1L) .name("JOKER House") .address("Seoul") .build(); given(restaurantService.getRestaurant(1004L)).willReturn(restaurant);
ResultActions resultActions = mockMvc.perform(get("/restaurants/1004"));
resultActions .andExpect(status().isOk()) .andExpect(content().string(containsString("\"id\":1004"))) .andExpect(content().string(containsString("\"name\":\"JOKER House\""))); }
|
특정 레스토랑을 가져오는 Service로직
RestaurantRepository 객체를 이용해 DB로부터 id값이 일치하는 레스토랑 정보를 가져온다. 만약 해당되는 Restaurant정보가 없다면 RestaurantNotFoundException
을 발생시킨다.
@Service @RequiredArgsConstructor public class RestaurantService { private final RestaurantRepository restaurantRepository;
public List<Restaurant> getRestaurants() { List<Restaurant> restaurants = restaurantRepository.findAll(); return restaurants; }
public Restaurant getRestaurant(Long id) { Restaurant restaurant = restaurantRepository.findById(id) .orElseThrow(() -> new RestaurantNotFoundException(id));
return restaurant; } }
|
Service로직 테스트코드 구현
특정 레스토랑정보를 정상적으로 가져오는 경우와 특정 레스토랑정보를 못가져오는 경우 두가지에 대한 테스트 코드를 작성해야 한다.
@Test public void 특정_레스토랑을_가져온다() { Restaurant restaurant = restaurantService.getRestaurant(1004L);
verify(restaurantRepository).findById(eq(1004L));
assertThat(restaurant.getId()).isEqualTo(1004L); }
@Test public void 없는_레스토랑을_가져온다() { assertThatThrownBy(() -> { Restaurant restaurant = restaurantService.getRestaurant(404L); }).isInstanceOf(RestaurantNotFoundException.class); }
|
public class RestaurantNotFoundException extends RuntimeException{ public RestaurantNotFoundException(Long id) { super("Could not find Restaurant " + id ); } }
|
@ControllerAdvice public class RestaurantErrorAdvice {
@ResponseBody @ResponseStatus(HttpStatus.NOT_FOUND) @ExceptionHandler(RestaurantNotFoundException.class) public String handleNotFound(){ return "{}"; } }
|