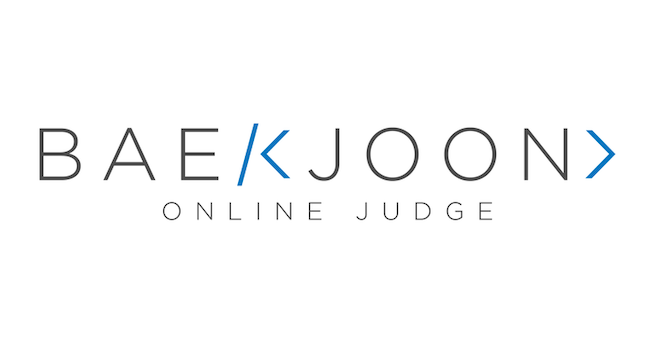
https://www.acmicpc.net/problem/1181
백준 3015 단어 정렬
문제 풀이
자료구조 Set을 사용하기 좋은 문제이다. 다만 Set에서 사용하는 정렬방식을 조건에 맞게 변형해줄 필요가 있다.
소스코드
#include <bits/stdc++.h> using namespace std;
set<pair<int, string>> s; int n;
int main(void) { cin.tie(0); cout.tie(0); ios_base::sync_with_stdio(false); cin >> n; while (n--) { string word; cin >> word; s.insert({word.length(), word}); }
for (auto iter = s.begin(); iter != s.end(); iter++) { cout << iter->second << '\n'; }
return 0; }
|
자바 소스 코드
Comparator 인터페이스를 이용해 set의 정렬기준을 사용자가 정의한 정렬기준으로 변경할 수 있다. Comparator 인터페이스를 사용하게 되면 compare 메소드를 오버라이딩해 원하는 정렬 기준을 적용할 수 있다.
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.Comparator; import java.util.Set; import java.util.TreeSet;
public class Main { public static void main(String args[]) throws IOException { BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int count = Integer.parseInt(br.readLine()); Set<String> words = new TreeSet<String>(new Comparator<String>() {
@Override public int compare(String o1, String o2) { if (o1.length() < o2.length()) { return -1; } else if (o1.length() > o2.length()) { return 1; } else { return o1.compareTo(o2); } } });
for (int countIdx = 0; countIdx < count; countIdx++) { String word = br.readLine();
words.add(word); }
words.stream().forEach(x -> System.out.println(x)); } }
|
파이썬 소스 코드
파이썬에서는 sorted 메소드에 정렬 기준을 넘겨주면 해당 정렬 기준을 이용해 정렬을 하게 된다.
n = int(input())
s = set()
for i in range(n): s.add(input())
s = sorted(sorted(s), key=len) for a in s: print(a)
|