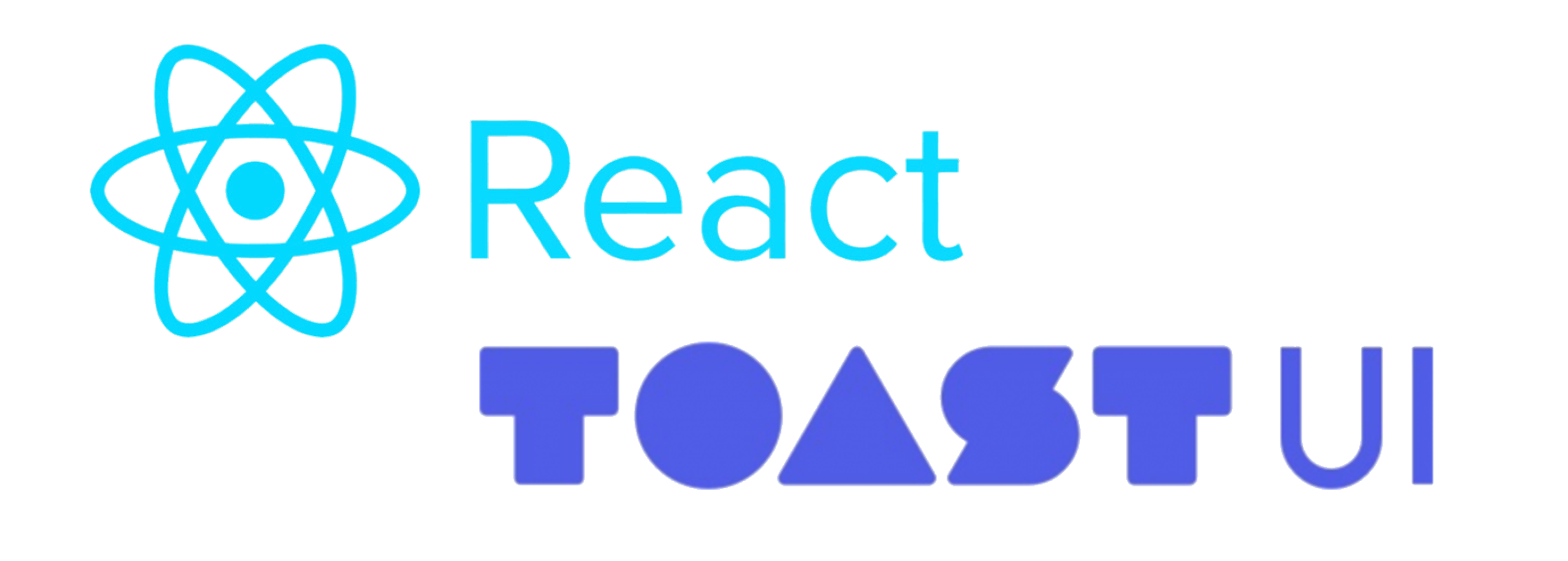
React Toast UI Editor 3 - 입력 내용 가져오기
Editor에 입력된 내용을 저장하고 관리하기 위해서는 입력된 값을 받아와야 한다. Toast UI Editor에서는 useRef를 이용해
const editorRef = useRef();
|
버튼 Event Handler 만들기
버튼을 만들어줘 버튼을 눌렀을 때 Editor에 입력된 내용을 반환해 주는 Evnet를 작성한다. 입력된 값을 받아올 때는 Ref 객체인 editorRef
를 이용해 Toast UI Editor 객체를 가져와 입력된 값을 받아올 수 있도록 한다.
const handleClick = () => { console.log(editorRef.current.getInstance().getMarkdown()); };
|
Button 클릭에 대한 이벤트 핸들러와 Editor 컴포넌트에 Ref 객체를 넣어준다.
<div> <Editor initialValue="hello react editor world!" previewStyle="vertical" height="600px" initialEditType="markdown" useCommandShortcut={true} ref={editorRef} plugins={[codeSyntaxHighlight]} /> <button onClick={handleClick}>Markdown 반환하기</button> </div>
|
전체 소스
import React, { useRef } from "react";
import { Editor } from "@toast-ui/react-editor"; import "@toast-ui/editor/dist/toastui-editor.css";
import codeSyntaxHighlight from "@toast-ui/editor-plugin-code-syntax-highlight/dist/toastui-editor-plugin-code-syntax-highlight-all.js";
import "prismjs/themes/prism.css";
const Write = () => { const editorRef = useRef();
const handleClick = () => { console.log(editorRef.current.getInstance().getMarkdown()); };
return ( <div> <Editor initialValue="hello react editor world!" previewStyle="vertical" height="600px" initialEditType="markdown" useCommandShortcut={true} ref={editorRef} plugins={[codeSyntaxHighlight]} /> <button onClick={handleClick}>Markdown 반환하기</button> </div> ); };
export default Write;
|
Editor에 입력된 값들이 Markdown 반환하기 버튼을 누를 때마다 Console에 찍히는 것을 확인할 수 있다.
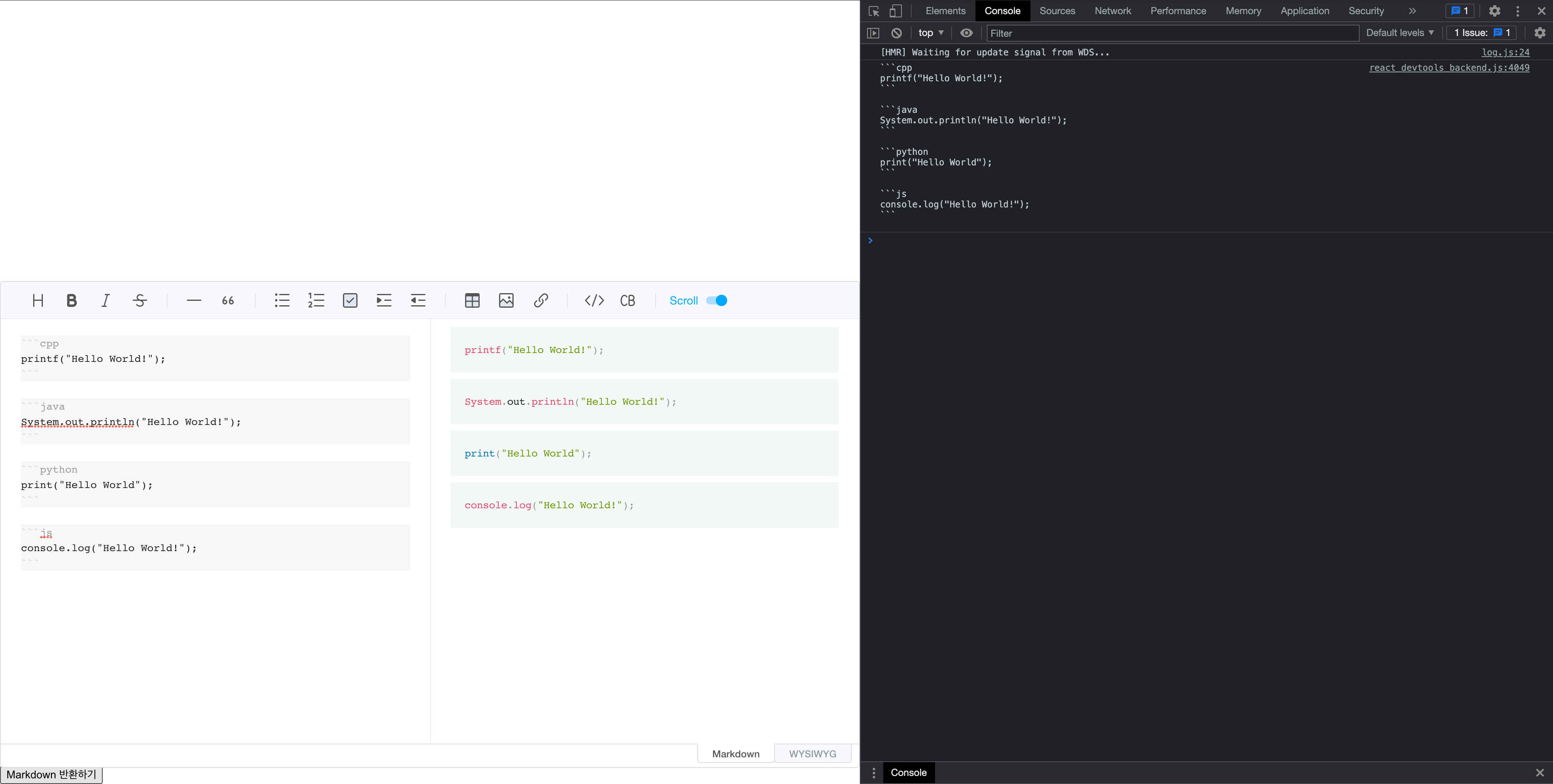
https://github.com/nhn/tui.editor/tree/master/apps/react-editor