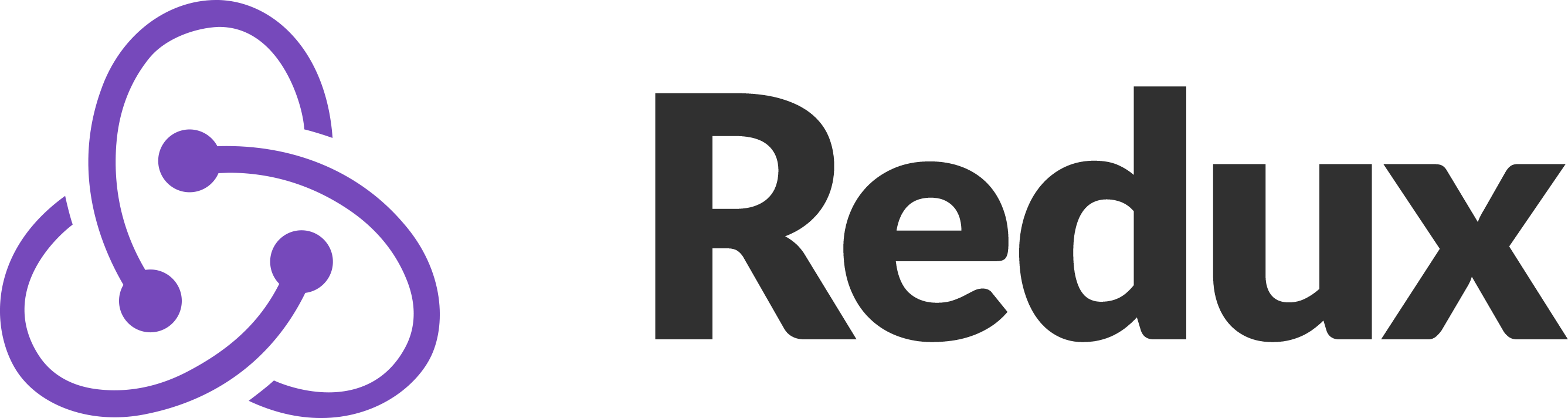
목차
Redux 사용하기
- 하나의 애플리케이션 안에는 하나의 Store 가 존재
- State 는 읽기 전용
- 불변성 을 지켜줘야 한다.
- 객체의 경우 Spread 연산자 를 사용해 기존 객체를 덮어써준다.
- 배열의 불변성을 지켜주는 내장함수를 사용해야 한다.
- 불변성을 지켜야만 Component 들이 제대로 Rerendering 된다.
- 변화를 일으키는 함수, Reducer 는 순수한 함수 여야 한다.
- Reducer 는 이전 상태 와 Action 객체 를 파라미터로 받는다.
- 이전 상태를 변경하지 않고 새로운 상태를 만들어 반환한다.(불변성 유지)
- 똑같은 파라미터로 호출된 리듀서는 언제나 똑같은 결과값을 반환해야 한다.
Redux 모듈 설치
Action
상태의 변화가 필요할 경우 Action 을 일으킨다.
- type 이라는 값이 필수적 있어야 한다.
- type 외에도 다른 값을 넣어줄 수 있다.
{ type: "INCREASE", data: { id: 0, text: "값 증가" } }
|
Action 생성 함수
Action 을 만들어 반환 하는 함수
- 파라미터를 받아와 Action 을 생성해주는 함수
- Action 생성함수를 만드는게 필수적이지는 않다.
const increase = () => ({ type: INCREASE })
const changeText = text => ({ type: CHANGE_TEXT, text });
|
Reducer
Action type 을 이용해 변화를 일으키는 함수
- state 와 action 2가지 파라미터를 가져온다.
- action type 을 이용해 데이터 업데이트를 진행한 후 새로운 state 를 반환한다.
- reducer 에서는 불변성을 유지해야 하기 때문에 state 가 객체 상태로 넘어오면 spread 연산자를 활용한다.
- default에서는 기존의 state를 반환하는 형태로 작성한다.(여러개의 리덕스를 합처 Root Reducer를 만들 수 있기 때문에)
function reducer(state = initialState, action) { switch (action.type) { case INCREASE: return { ...state, counter: state.counter + 1 } case DECREASE: return { ...state, counter: state.counter - 1 } case CHANGE_TEXT: return { ...state, text: action.text } case ADD_TO_LIST: return { ...state, list: state.list.concat(action.item) } default: return state; } }
|
Store
State 를 관리하는 저장소로 보통은 하나의 Application 당 하나의 Store 를 만든다.
현재 앱의 상태 , Reducer , 내장 함수 들을 가지고 있다.
- dispatch (내장 함수)
- dispatch 는 action 을 발생시킨다.
- 발생 시킨 action 은 Reducer 로 전달돼 새로운 상태를 반환한다.
- subscribe (내장 함수)
- 파라미터로 함수를 넣어주면 action 이 dispatch 될 때마다 함수가 호출 된다.
import { createStore } from 'redux';
const store = createStore(reducer);
|
store.dispatch(increase()); store.dispatch(decrease()); store.dispatch(changeText('안녕하세요')); store.dispatch(addToList({ id: 1, text: '와우' }));
|
React Redux 적용
react-redux
패키지의 Provider 를 이용해 store 를 등록한 후 사용하면 된다.
import React from 'react'; import ReactDOM from 'react-dom'; import './index.css'; import App from './App'; import reportWebVitals from './reportWebVitals'; import { createStore } from 'redux'; import rootReducer from './modules'; import { Provider } from 'react-redux';
const store = createStore(rootReducer);
ReactDOM.render( <Provider store={store}> <App /> </Provider>, document.getElementById('root') );
reportWebVitals();
|