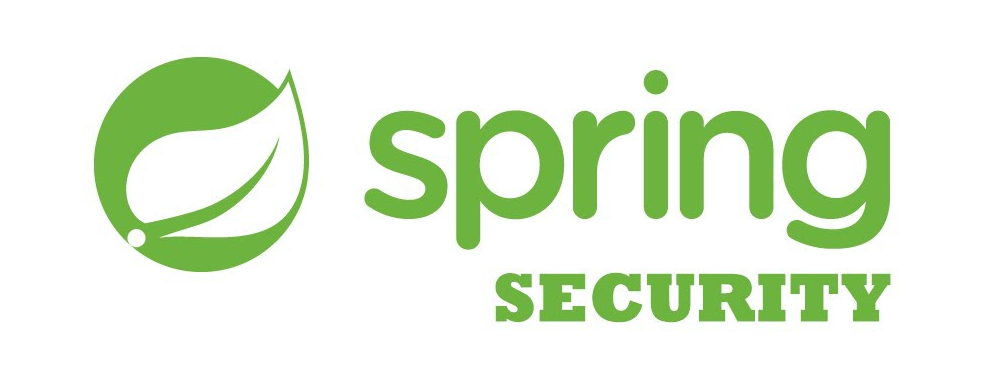
목차
Controller
@RestController public class HelloController {
@GetMapping("/hello") public String hello(){ return new String("hello"); } }
|
@WithMockUser
@WithMockUser 는 Security Test 를 진행할 때 인증된 Authentication 객체를 만들어 인증된 상태로 Test 를 진행할 수 있게 도와준다.
- 속성
- username : Authentication 객체에서 사용할 username 을 설정
- password : Authentication 객체에서 사용할 password 을 설정
- roles : Authentication 객체에서 사용할 role 을 설정
- authorities : Authentication 객체에서 사용할 authorities 을 설정
@WebMvcTest @AutoConfigureMockMvc class HelloControllerTest { @Autowired MockMvc mockMvc;
@Test @WithMockUser(username = "user", password = "1234") void mockUserTest() throws Exception { mockMvc.perform(get("/hello")) .andDo(print()) .andExpect(status().isOk()); } }
|
@WithMockUser 권한 사용 하기
Security Config 추가하기
Resource 에 각각 다른 권한 별로 접근이 가능하도록 설정한다.
@Override protected void configure(HttpSecurity http) throws Exception { http.authorizeRequests() .antMatchers("/hello").hasRole("USER") .antMatchers("/manager").hasRole("MANAGER") .anyRequest() .authenticated(); }
|
Controller 추가
@GetMapping("/manager") public String manager(){ return "Hello Manager"; }
|
테스트 코드 작성
@WithMockUser 를 통해 생성된 Authentication 객체는 role 이 USER
기 때문에 MANAGER
권한이 필요한 resource 에는 접근할 수 없다.
@Test @WithMockUser(username = "user", password = "1234", roles = "USER") void mockManagerTest() throws Exception { mockMvc.perform(get("/manager")) .andDo(print()) .andExpect(status().isForbidden()); }
|
@WithAnonymousUser
@WithAnonymousUser 는 anonymous 사용자로 테스트를 진행한다.
@Test @WithAnonymousUser void mockAnonymousUserTest() throws Exception { mockMvc.perform(get("/hello")) .andDo(print()) .andExpect(status().isForbidden()); }
|